Using a plugin as an effect
All custom Videosync plugins are audio effects. Some plugins, like Lines, don't use input, effectively acting as instruments, you probably want to use them only at the beginning of the device chain of a MIDI track. But a plugin can just as well be used as a video effect, mixed with other video effects.
To develop a plugin that uses the track content or the output of a previous plugin, you need to actively involve the incoming image. Let's say we want the Better Lines
plugin we created in the previous section to show the incoming image in a circle within the lines image. We could mix it in depending on the distance from the center.
Replace the last line of the shader with the following block:
vec4 linesResult = vec4(red, green, blue, alpha);
vec4 incoming = IMG_THIS_PIXEL(inputImage);
float dist = distance(vec2(x, y), vec2(0, 0));
gl_FragColor = mix(incoming, linesResult, smoothstep(0.2, 0.3, dist));
Not just RGB but also the alpha of the incoming texture is mixed with the alpha of the result. This is good practice for an effect since the incoming image may contain useful alpha information.
The result of Lines should now look like this (e.g. added to a track that plays Beeple's domechards):
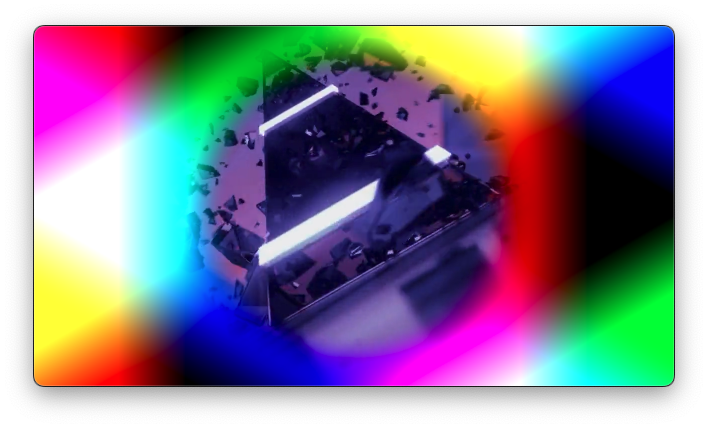
(Not pretty? We're sure you can do better!)
For more information, the Plugin SDK contains examples of plugins that act as an effect. Also, the complete list of options you have at your disposal in your plugin code can be found in the ISF specification.
Alpha (Opaqueness)
Contrary to what you might expect, using alpha is not the way to make the image of the previous plugin visible through the current result.
In the output of the Lines plugin, the alpha (synonym for opaqueness) is always 1
:
float alpha = 1;
gl_FragColor = vec4(red, green, blue, alpha);
Normally, a lower alpha value makes the result more transparent.
Contrary to what you might expect, in Videosync transparency does not mix the output of a plugin with the output of the previous plugin. The alpha channel is only used when combining a track or rack chain's final output with others. This can depend on the blend mode. For more info, see Blending.
So we want plugins to manipulate the alpha channel rather than consume it, which would be the case if the plugin results would blend with the previous plugin.
For more in-depth info about custom plugins, such as using vertex shaders, multiple passes and distributing plugins, read on!